BY KADDARK NEWFANY VARAWIBWUB CHEESECAKE PIZZAVAMPIRE
RESTful API คืออะไร
เอาไว้สร้าง Web Service แบบเรียบง่าย โดยเรียกใช้ผ่านทาง HTTP Method GET / POST / PUT / DELETE และส่งข้อมูลออกมาในรูปของ XML หรือแบบ JSON ทำให้ปริมาณข้อมูลที่รับส่ง น้อยกว่าการใช้ Protocol SOAP อยู่มาก
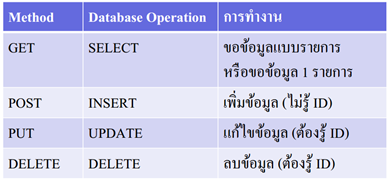
ประโยชน์ของ RESTful API
— ทำการอยู่บน HTTP และทำตามมาตรฐานของ HTTP จึงทำให้พัฒนาได้ง่าย
— สนับสนุนรูปแบบข้อมูลมากมาย เช่น XML, JSON, Plain Text และอื่น ๆ อีกมากมาย
— รองรับเรื่อง caching ข้อมูล
— รองรับการขยายระบบได้ง่าย
— Stateless ทำงานโดยไม่ต้องมี session
— สนับสนุนรูปแบบข้อมูลมากมาย เช่น XML, JSON, Plain Text และอื่น ๆ อีกมากมาย
— รองรับเรื่อง caching ข้อมูล
— รองรับการขยายระบบได้ง่าย
— Stateless ทำงานโดยไม่ต้องมี session
ขั้นตอนที่ 1 คือ
การสร้าง Database ขึ้นมา สามารถดาวโหลด
ได้ที่ https://raw.githubusercontent.com/bahar/WorldCityLocations/master/World_Cities_Location_table.sql นำโค๊ดทั้งหมดในเว็บนี้ไปใส่ notepad ตั้งชื่อ location.sql แล้วทำการ import เข้า http://localhost/phpmyadmin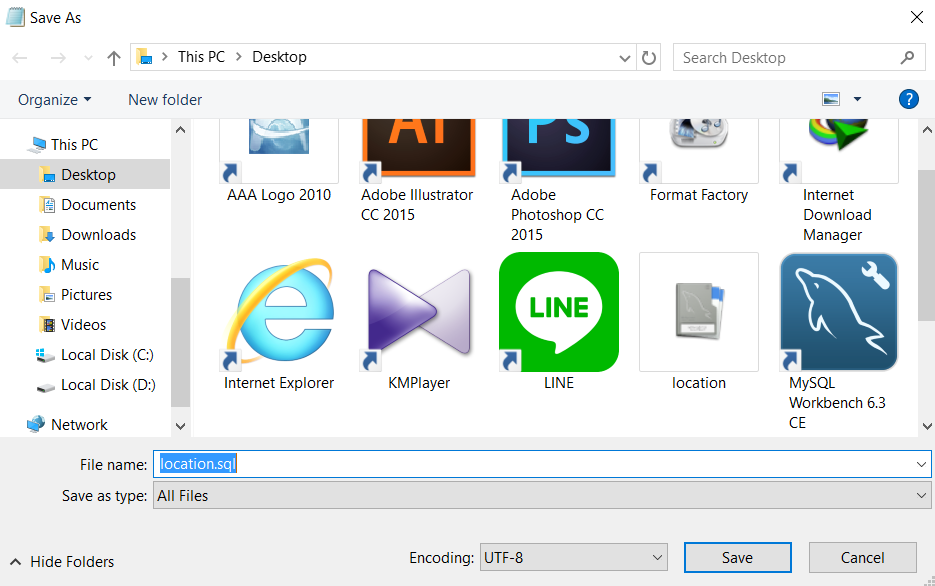
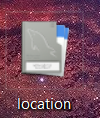
หลังจากได้ DB มาแล้ว ทำการ import database เข้าไป
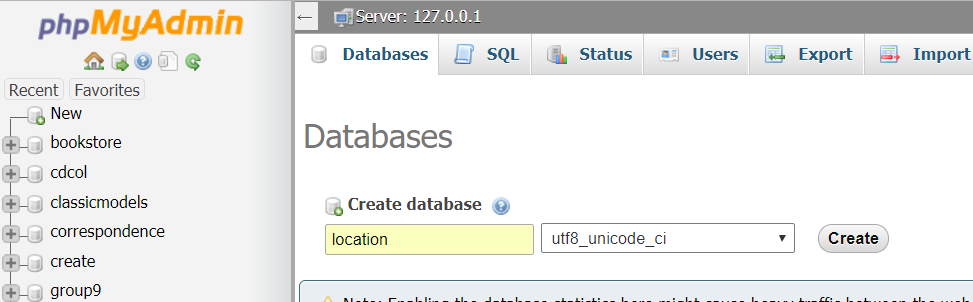
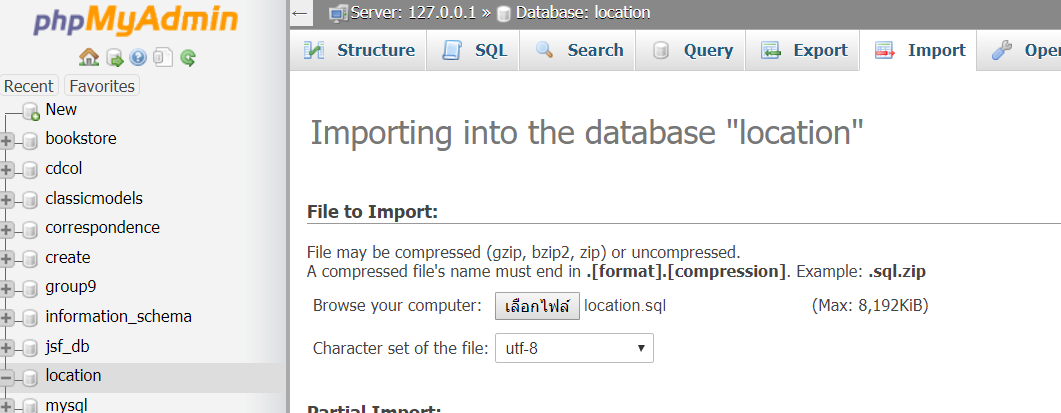
ขั้นตอนที่ 2 คือ
อย่าลืม !!! เปลี่ยน database นะ ในโฟลเดอร์ config ไฟล์ db.php เปลี่ยน database เป็น location ด้วย
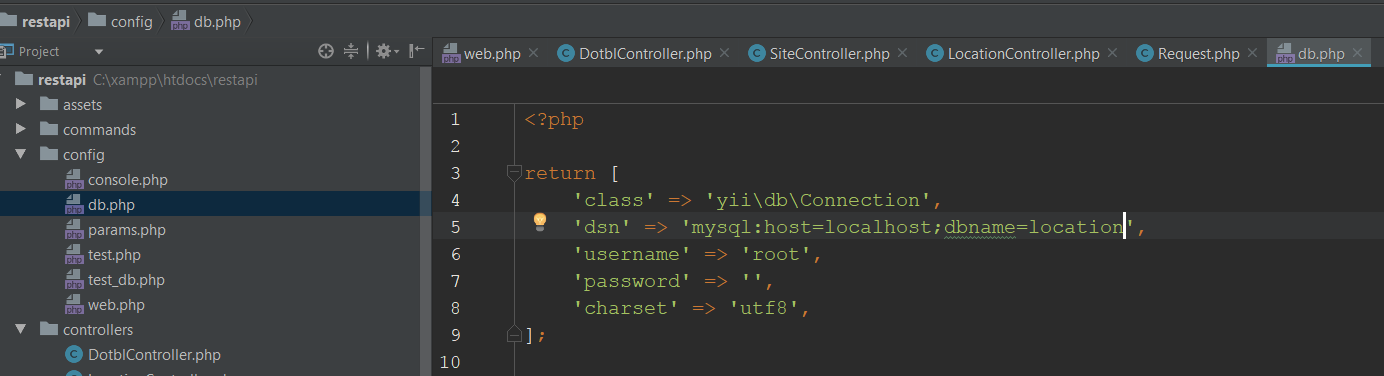
สร้าง model โดยใช้ gii generator เพื่อให้ง่ายต่อการทำควรทำ pretty url ก่อน ตามเว็บ http://dixonsatit.github.io/2014/11/30/modrewrite.html นี้เลย
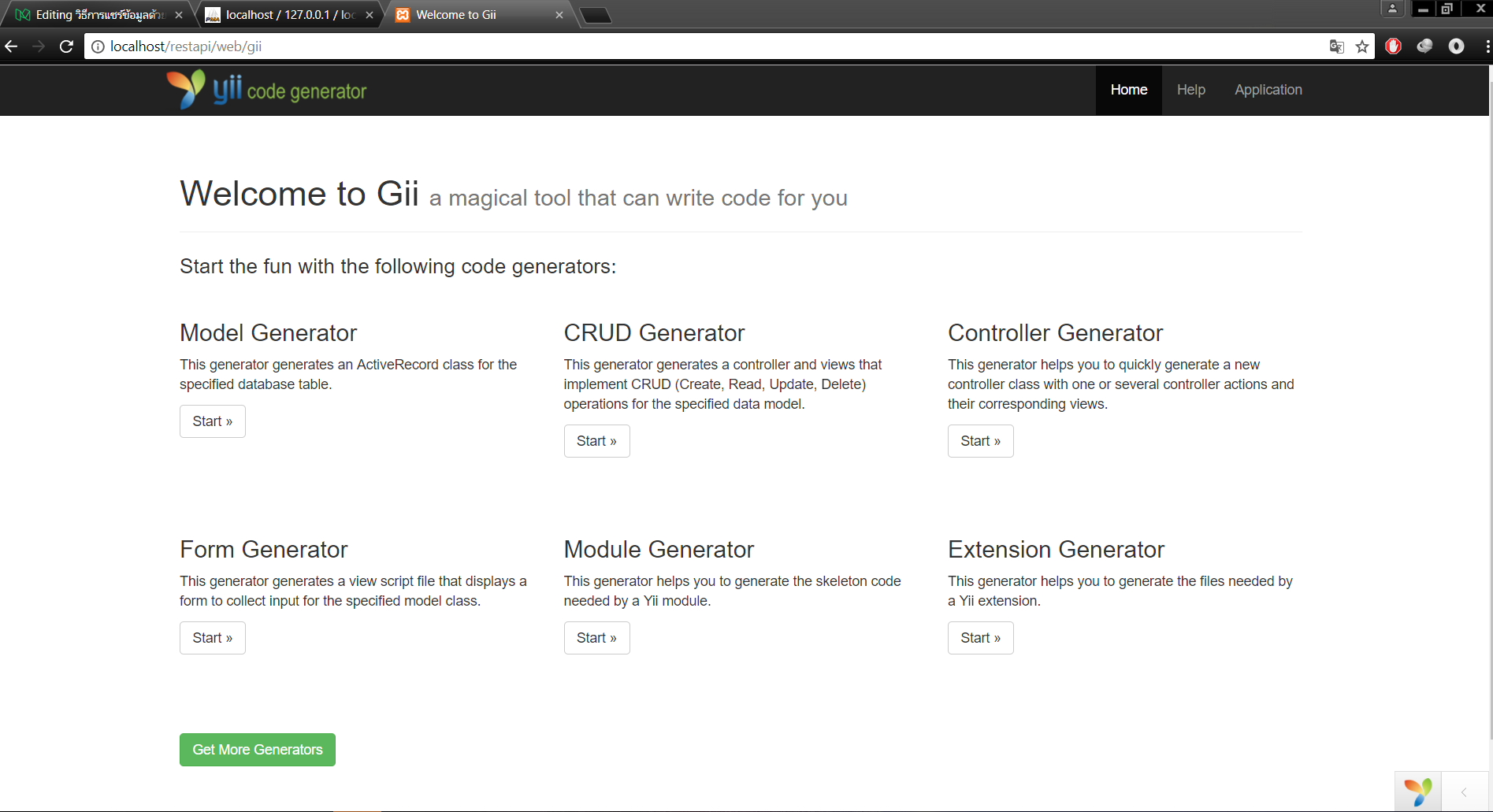
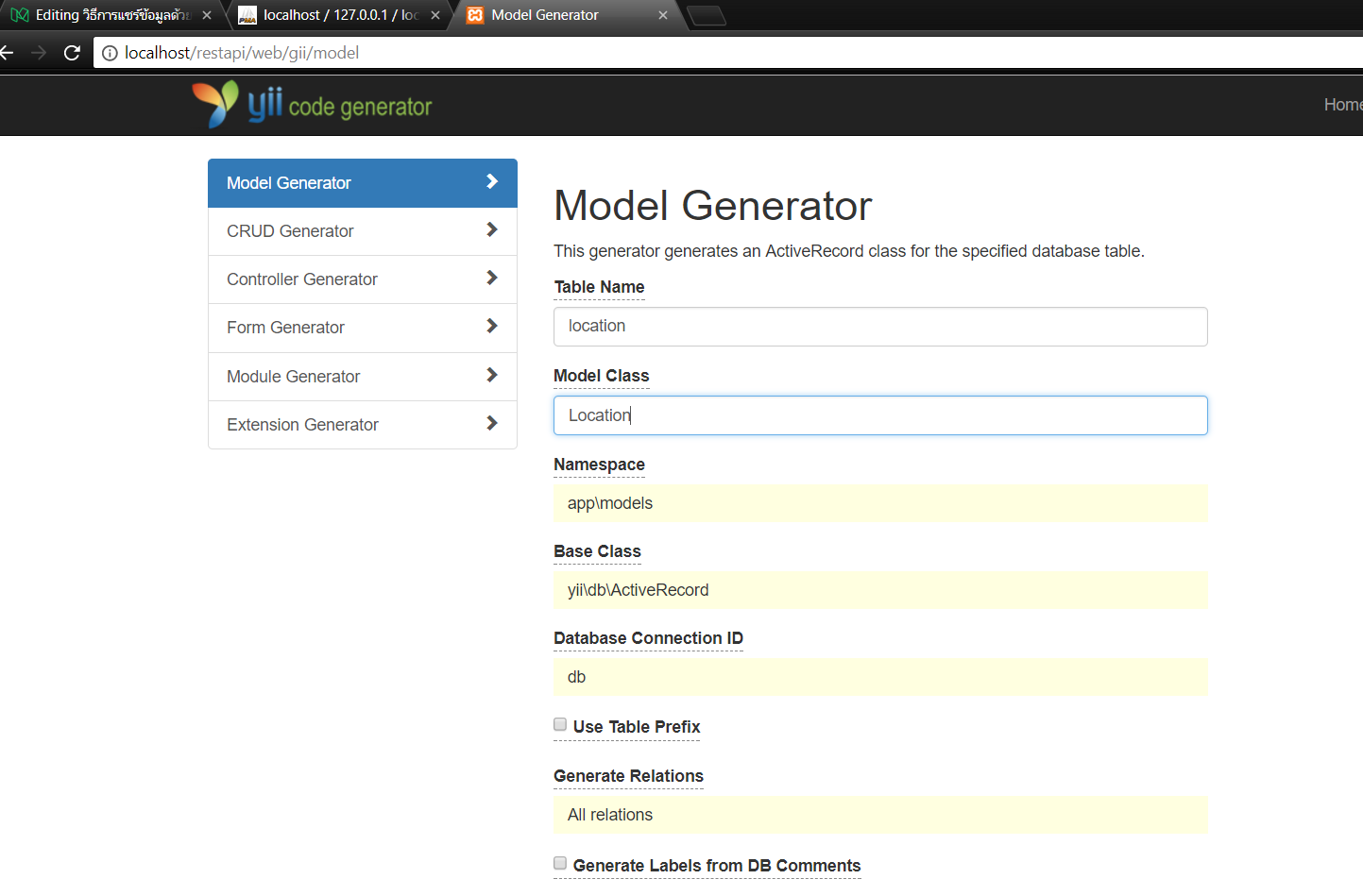


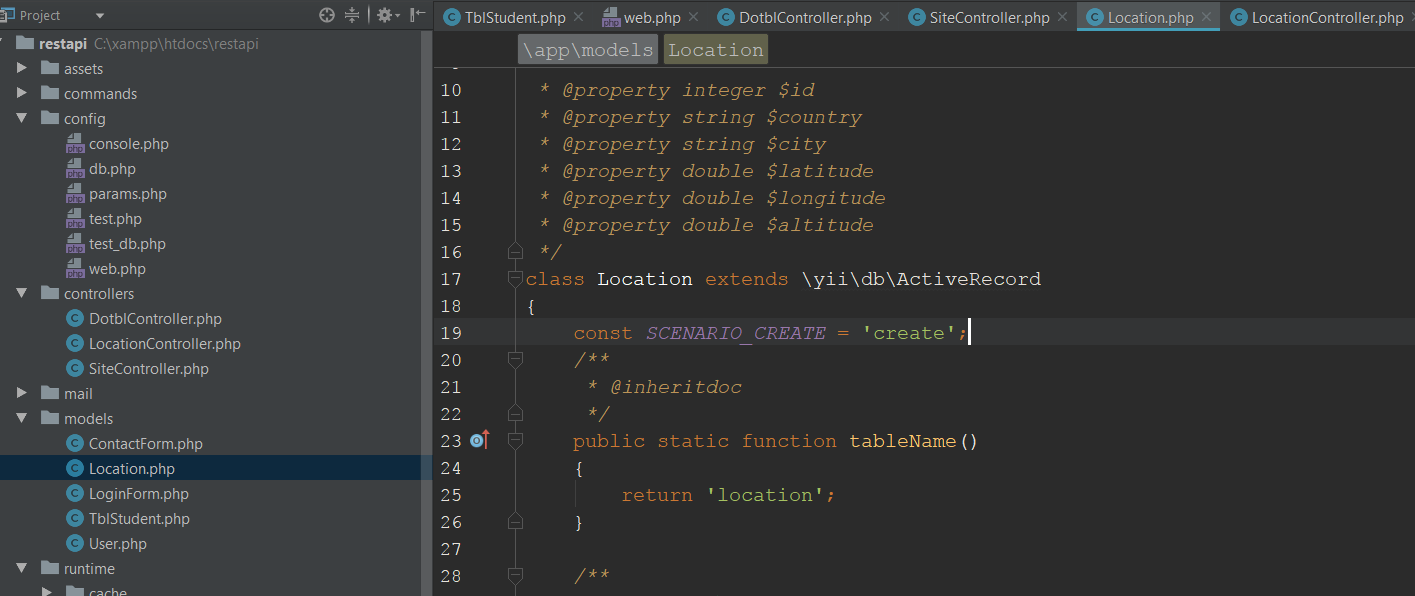
ในไฟล์ models : Location.php เพิ่มคำสั่ง
const SCENARIO_CREATE = ‘create’;public function scenarios()
{
$scenarios = parent::scenarios();
$scenarios[‘create’] = [‘id’,’country’,’city’,’latitude’,’longitude’,’altitude’]; //ต้องเหมือนใน databasereturn $scenarios;
}
จะได้ไฟล์ Location.php ดังนี้
<?php namespace app\models; use Yii; /** * This is the model class for table "location". * * @property integer $id * @property string $country * @property string $city * @property double $latitude * @property double $longitude * @property double $altitude */class Location extends \yii\db\ActiveRecord { const SCENARIO_CREATE = 'create'; /** * @inheritdoc */ public static function tableName() { return 'location'; } /** * @inheritdoc */ public function rules() { return [ [['country', 'city', 'latitude', 'longitude', 'altitude'], 'required'], [['latitude', 'longitude', 'altitude'], 'number'], [['country'], 'string', 'max' => 25], [['city'], 'string', 'max' => 40], ]; } public function scenarios() { $scenarios = parent::scenarios(); $scenarios['create'] = ['id','country','city','latitude','longitude','altitude']; //ต้องเหมือนใน database return $scenarios; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'country' => 'Country', 'city' => 'City', 'latitude' => 'Latitude', 'longitude' => 'Longitude', 'altitude' => 'Altitude', ]; } }
ขั้นตอนที่ 3 คือ สร้าง controller สำหรับจัดการ
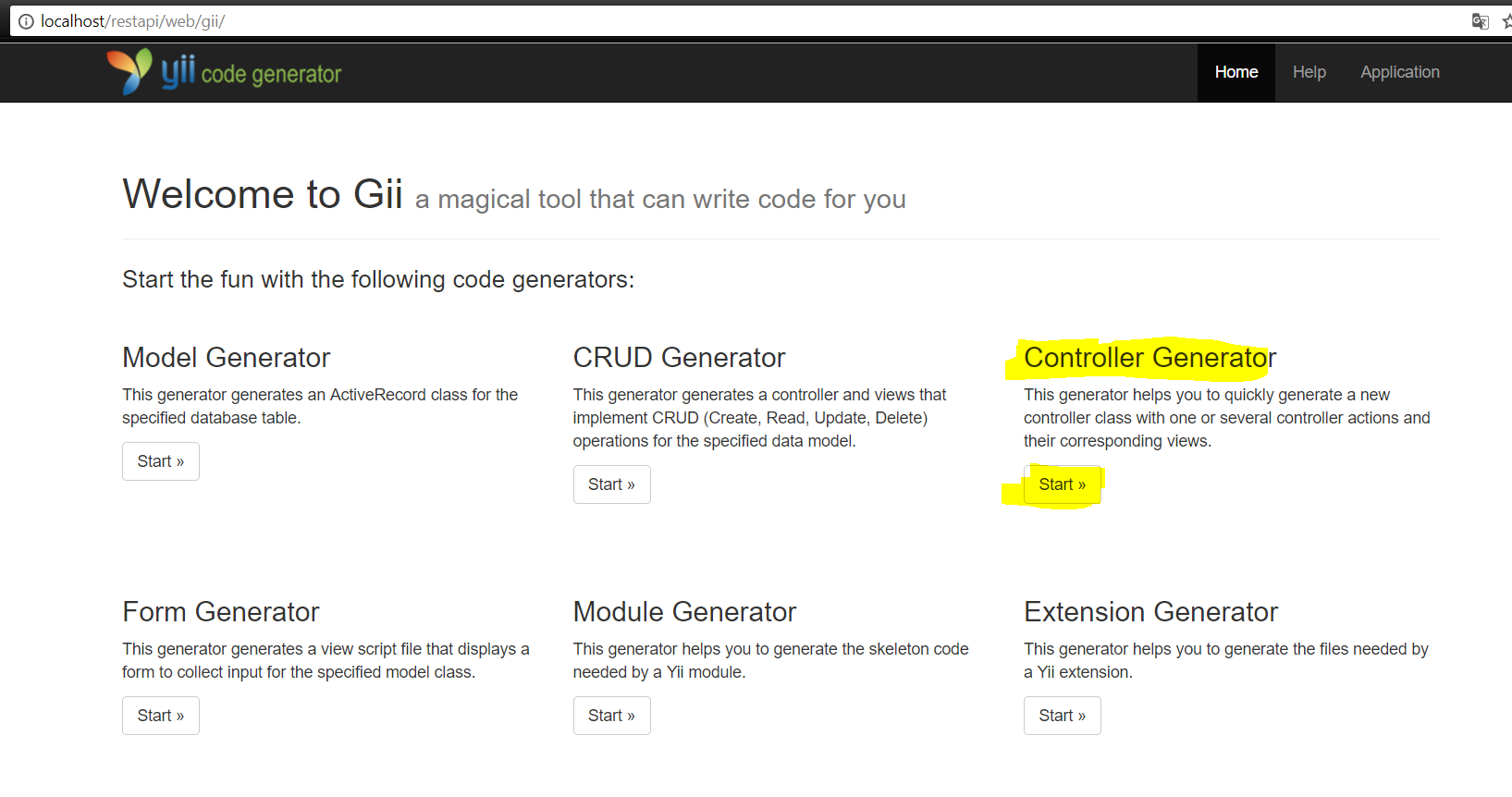


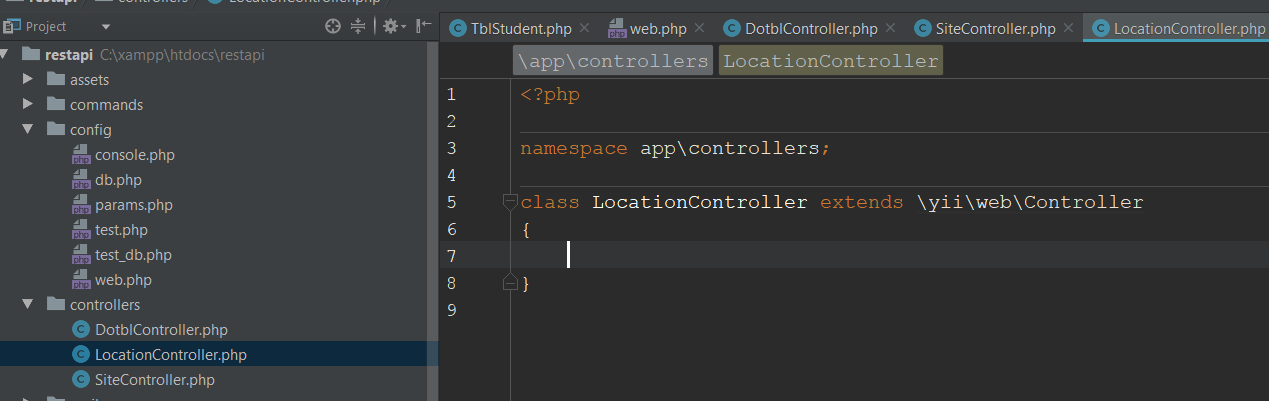
เพิ่มคำสั่งในไฟล์ LocationController.php ดังนี้
<?php
namespace app\controllers;
use app\models\Location;
use yii\filters\ContentNegotiator;
use yii\rest\ActiveController;
use yii\web\Response;
class LocationController extends ActiveController
{
public $enableCsrfValidation = false;
public function behaviors()
{
return [
[
'class' => ContentNegotiator::className(),
'only' => ['index', 'view'],
'formats' => [
'application/json' => Response::FORMAT_JSON,
],
],
];
}
public $modelClass = 'app\models\Location';
public $serializer = [
'class' => 'yii\rest\Serializer',
'collectionEnvelope' => 'items',
];
public function actionPost() //create
{
\Yii::$app->response->format = \yii\web\Response::FORMAT_JSON;
$location = new Location();
$location->scenario = Location::SCENARIO_CREATE;
$location->attributes = \yii::$app->request->post();
if ($location->validate()) {
$location->save();
return array('status' => true, 'data' => 'Record is successfully updated');
} else {
return array('status' => false, 'data' => $location->getErrors());
}
}
public function actionGet()
{
\Yii::$app->response->format = \yii\web\Response:: FORMAT_JSON;
$location = Location::find()->all();
if (count($location) > 0) {
return array('status' => true, 'data' => $location);
} else {
return array('status' => false, 'data' => 'No Location Found');
}
}
public function actionPut()
{
\Yii::$app->response->format = \yii\web\Response:: FORMAT_JSON;
$attributes = \yii::$app->request->post();
$location = Location::find()->where(['ID' => $attributes['id']])->one();
if (count($location) > 0) {
$location->attributes = \yii::$app->request->post();
$location->save();
return array('status' => true, 'data' => 'Location record is updated successfully');
} else {
return array('status' => false, 'data' => 'No Location Found');
}
}
public function actionDeleteRoll()
{
\Yii::$app->response->format = \yii\web\Response:: FORMAT_JSON;
$attributes = \yii::$app->request->post();
$location = Location::find()->where(['ID' => $attributes['id']])->one();
if (count($location) > 0) {
$location->delete();
return array('status' => true, 'data' => 'Location record is successfully deleted');
} else {
return array('status' => false, 'data' => 'No Location Found');
}
}
}
หลังจากนั้นเพิ่มคำสั่งเปิดการใช้งานเพื่อรับค่าที่อยู่ในรูปแบบของ Jsonใน ไฟล์ web.php ในโฟลเดอร์ config
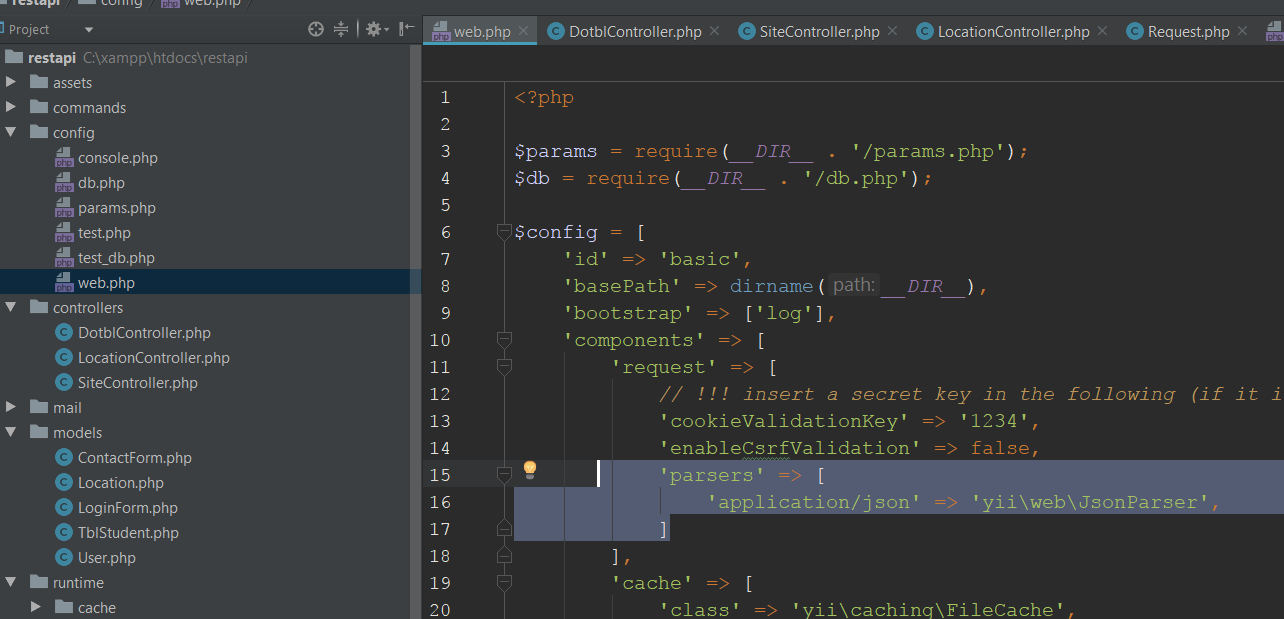
'parsers' => [
'application/json' => 'yii\web\JsonParser',
]
และอีกอย่างที่สำคัญคือลงตัวช่วยการแสดงผล json
สามารถทดสอบได้โดยการใส่ URL :
localhost/ชื่อโปรเจค/web/location
เช่นในตัวอย่างเป็น localhost/restapi/web/location
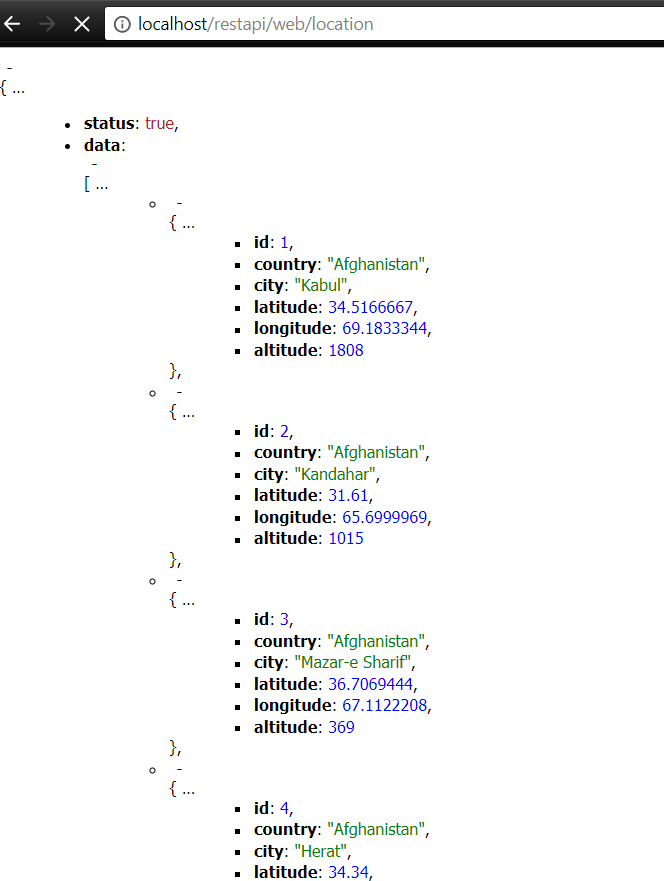
ขั้นตอนที่ 4
โหลดตัวช่วย Postman ช่วยทดสอบ API ว่าทำงานได้หรือไม่
สะดวกมากใช้ผ่าน chrome ได้เลยกดติดตั้งแล้วเปิดแอพขึ้นมาเลย

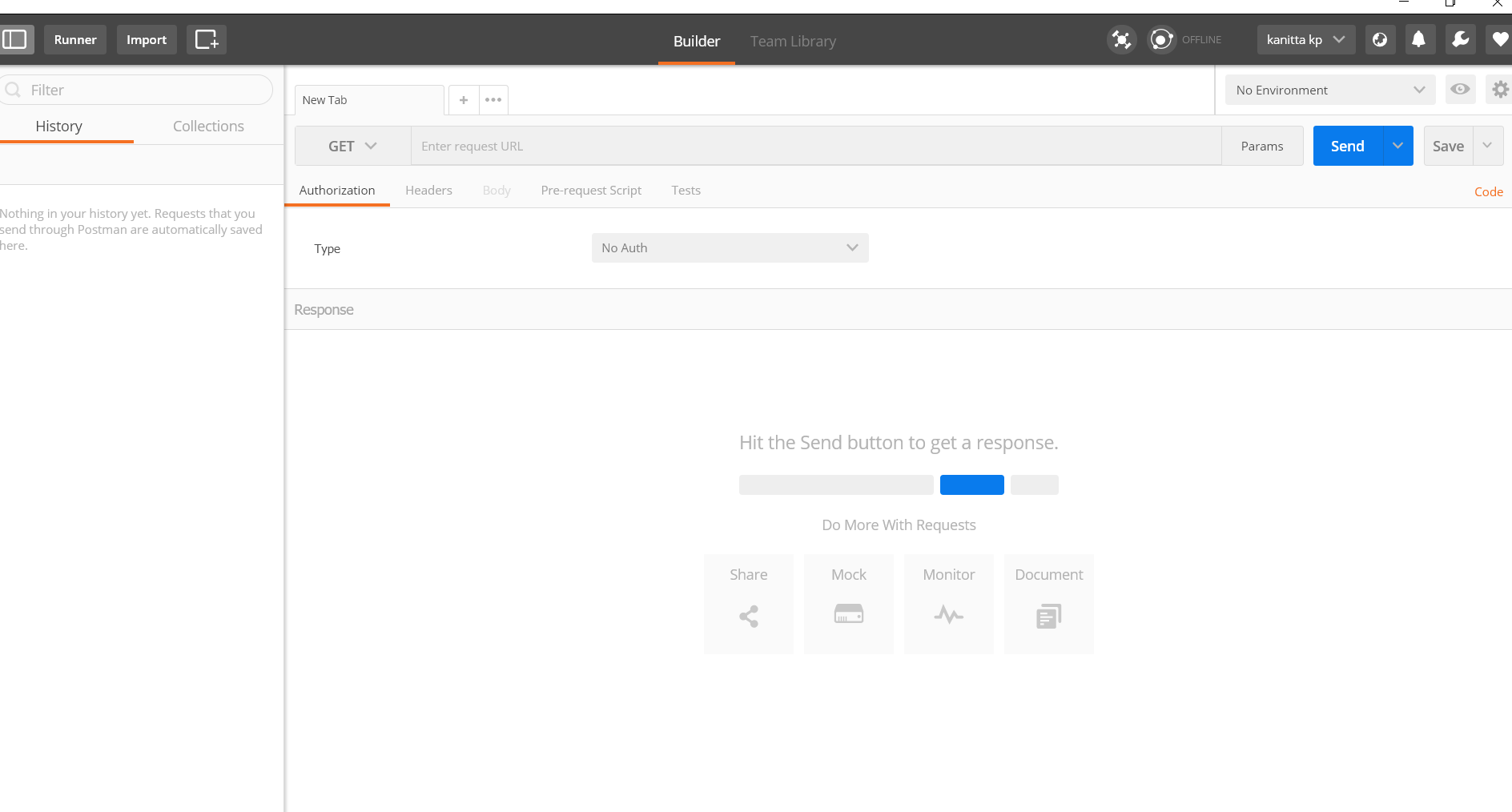
ขั้นตอนที่ 5 เริ่มใช้ postman กันเล๊ยยยย :]
GET
โดยใส่ URL: localhost/restapi/web/location เพื่อเรียก method get ใน controller ลงไปแล้วเลือกด้านหน้าในวงสีแดงเป็น get ด้วยนะ แล้วกด send ก็จะเป็นการ select ค่าทั้งหมดในตาราง location มาแสดงเป็น json ด้านล่าง
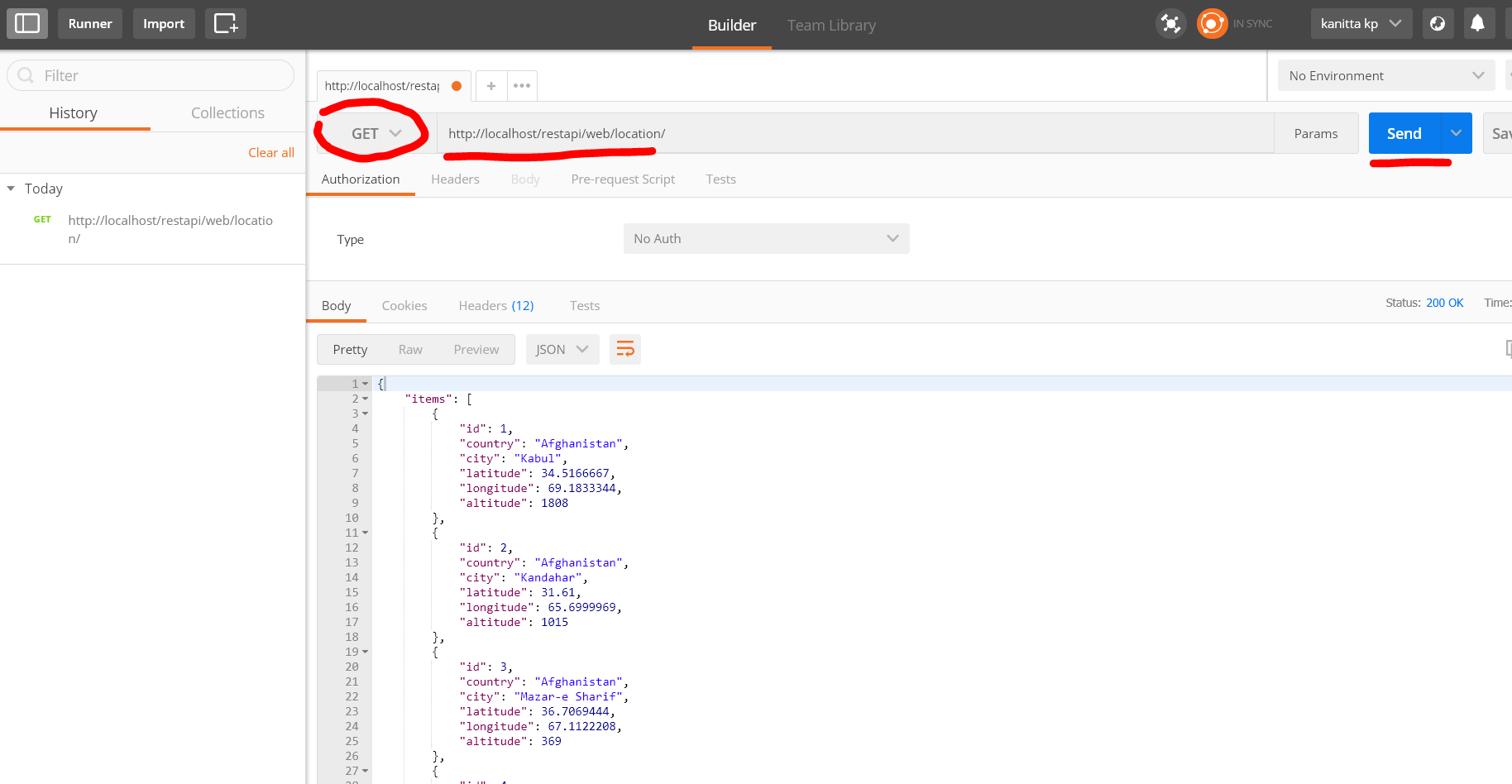
ถ้าใส่ต้องการค้นหาเฉพาะ id ก็ใส่ URL: localhost/restapi/web/location/1
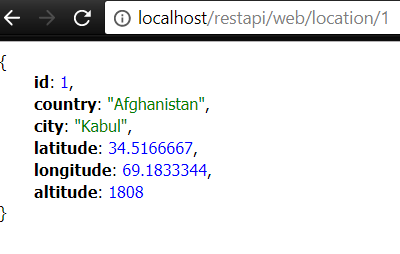
POST
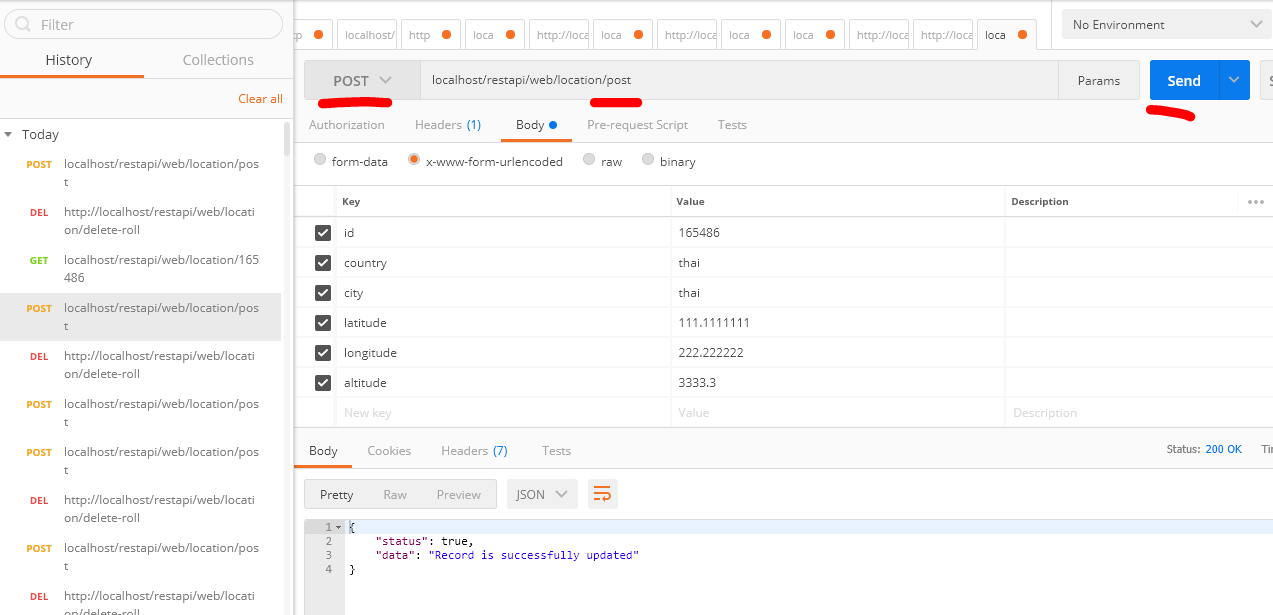
โดยใส่ URL : localhost/restapi/web/location/post
เลือก POST ด้านหน้า URL แล้วเพิ่ม attributes ตามตารางเติม value แล้วกดsend จะขึ้นว่าบันทึกแล้ว หรือตรวจสอบโดยเรียก
localhost/restapi/web/location/ 165486
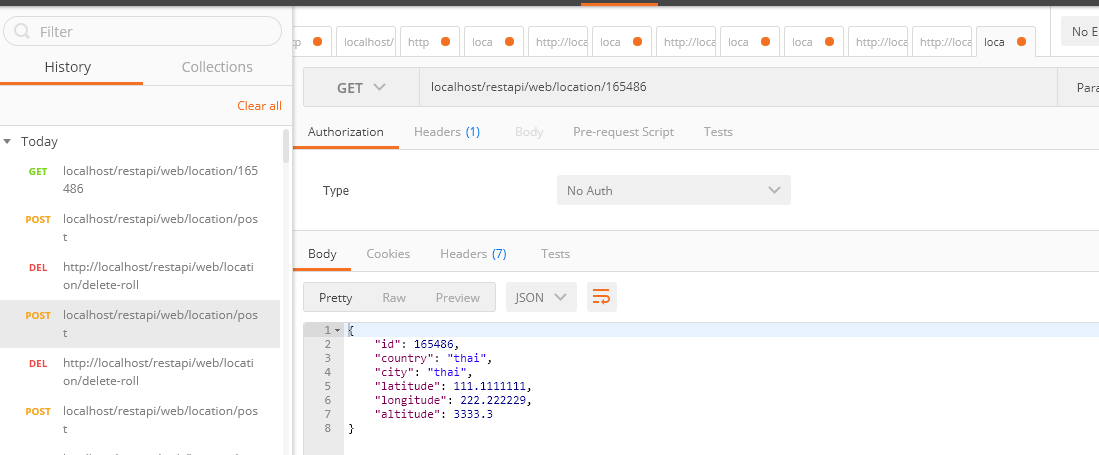
PUT หรือ UPDATE นั่นเอง
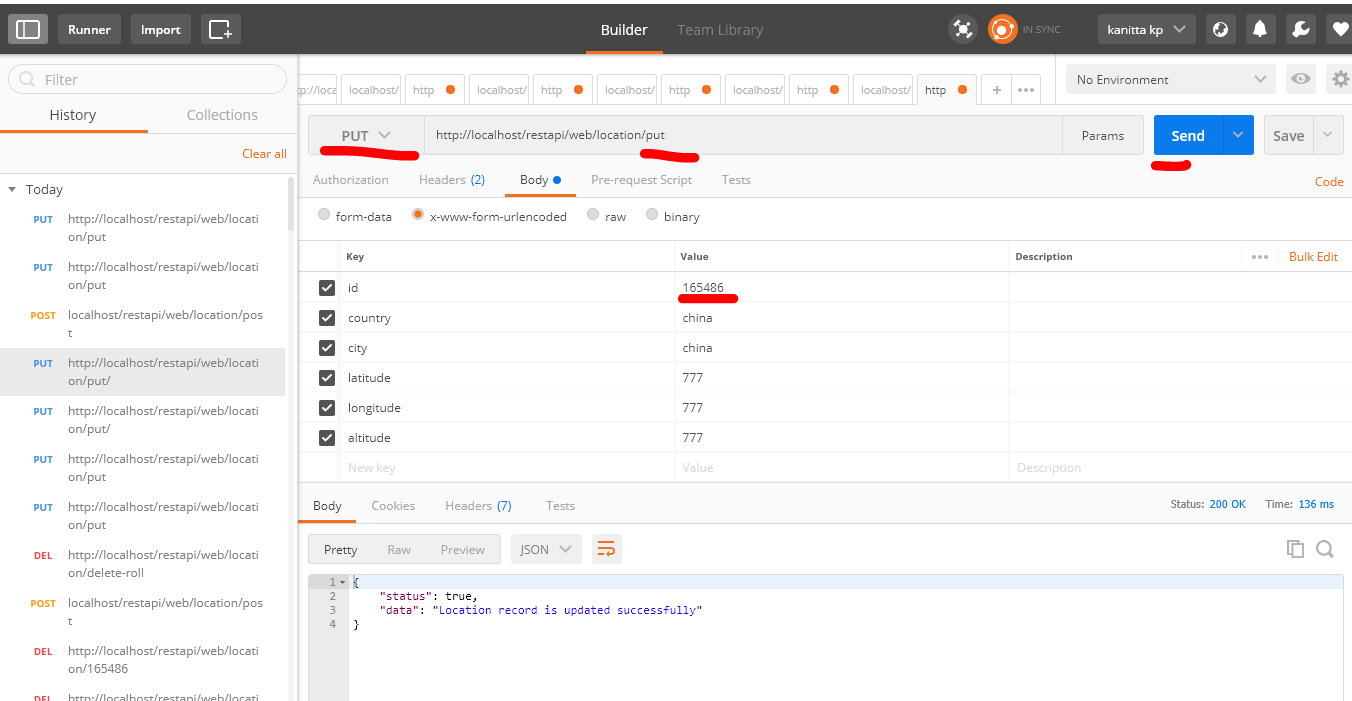


DELETE
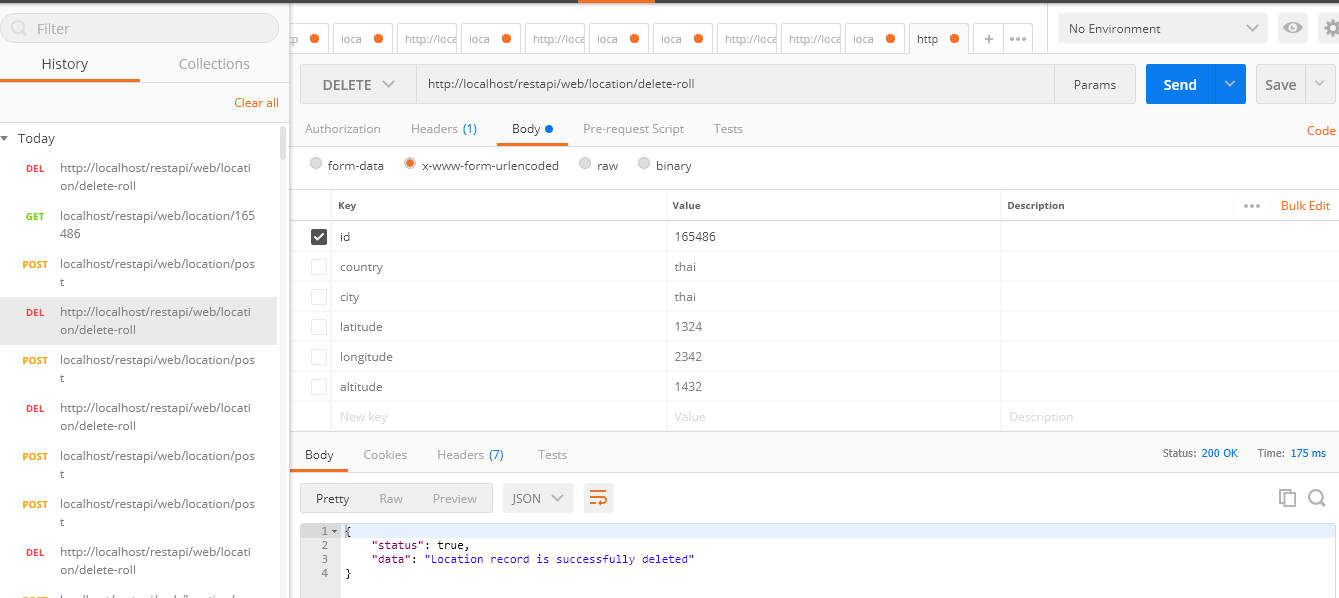
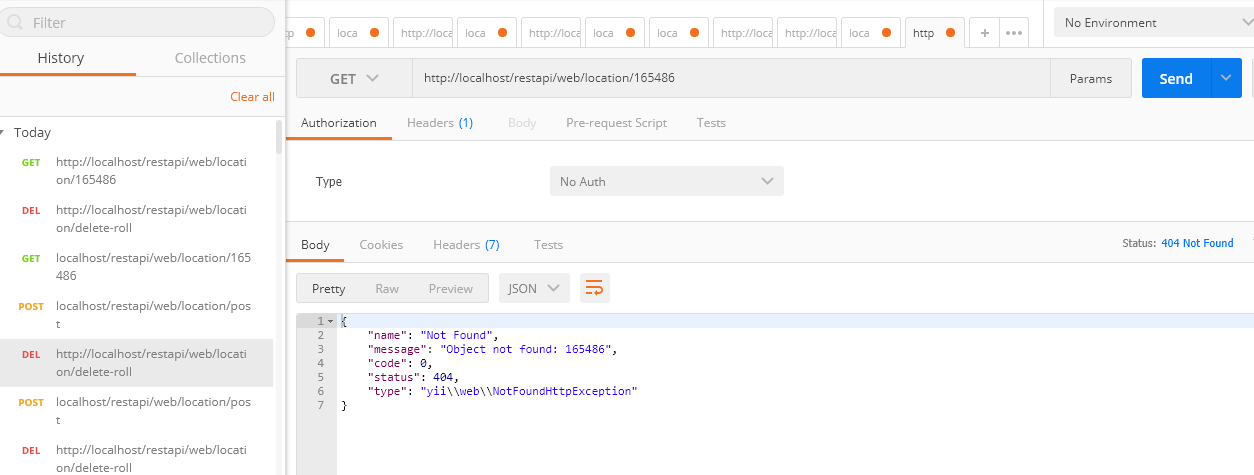
THANK YOU …. ^+++^
ไม่มีความคิดเห็น:
แสดงความคิดเห็น